Web development often involves interacting with APIs or fetching data from servers using Python’s powerful requests
library. Knowing how to pass parameters into a URL is crucial for ensuring your requests retrieve the correct information. This guide walks you through the steps, explains relevant concepts, and provides actionable examples to make the process seamless.
What Are URL Parameters?
URL parameters are pieces of information appended to a URL to modify the request or provide additional context. These parameters are typically included after a question mark (?
) and consist of key-value pairs separated by an equals sign (=
). For example:
https://api.example.com/data?key1=value1&key2=value2
Why Are URL Parameters Important?
- Customization: Tailor requests for specific data.
- Filtering: Narrow down search results.
- Pagination: Handle large datasets by splitting responses.
- Interactivity: Enable dynamic interactions with web services.
Basics of Python’s requests
Library
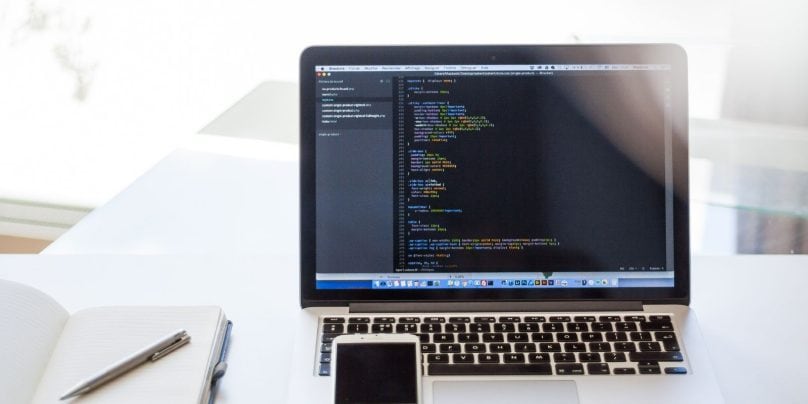
requests
is a user-friendly Python library for sending HTTP requests. With simple syntax and robust functionality, it’s a preferred choice for developers to interact with APIs and web services.
Understanding URL structure is vital for passing parameters effectively. A URL consists of:
- Base URL: The main address (e.g.,
https://api.example.com
). - Query String: A set of key-value pairs following a
?
(e.g.,?key1=value1
). - Fragment: Optional section after a
#
for client-side use.
How to Pass Parameters in GET
Requests
When using a GET
request, parameters are appended to the URL. Python’s requests
library simplifies this with the params
argument:
import requests
url = "https://api.example.com/data"
params = {"key1": "value1", "key2": "value2"}
response = requests.get(url, params=params)
print(response.url)
Output:
https://api.example.com/data?key1=value1&key2=value2
This approach ensures that parameters are encoded correctly.
Dynamic URL Building with Parameters
Dynamic URLs can be constructed by embedding variables:
base_url = "https://api.example.com/data"
query = {"search": "Python", "limit": 10}
response = requests.get(base_url, params=query)
This method is ideal for scenarios like filtering search results or handling dynamic queries.
Encoding Special Characters in Parameters
Special characters (e.g., spaces, &
, %
) must be URL-encoded. Python’s requests
library automatically handles this. For manual encoding, use urllib.parse.quote
:
from urllib.parse import quote
param = quote("value with spaces")
print(param) # Output: value%20with%20spaces
Advanced Techniques and Best Practices
Here are some tips from our side and good best practices to follow:
Using urllib
for URL Manipulation
Although requests
is the go-to library, Python’s urllib
offers additional flexibility for constructing and parsing URLs:
from urllib.parse import urlencode
base_url = "https://api.example.com/data"
params = {"key1": "value1", "key2": "value2"}
full_url = f"{base_url}?{urlencode(params)}"
print(full_url)
Handling Complex Parameters
For nested data structures or arrays, use JSON or encode the parameters:
params = {"filters": {"category": "books", "price": ["10", "20"]}}
response = requests.get(url, params=params)
Avoiding Common Errors
- Incorrect Encoding: Always verify special characters.
- Unnecessary Parameters: Avoid adding irrelevant keys.
- Missing Base URL: Ensure the base URL is correct.
Practical Examples and Use Cases
These are some examples that demonstrate how such scenarios simplify real-world API interactions:
Example 1: Making a Simple API Call
Retrieve weather data from an API:
url = "https://api.weather.com/v1"
params = {"location": "New York", "unit": "metric"}
response = requests.get(url, params=params)
print(response.json())
Example 2: Pagination with Query Strings
Handle paginated results:
for page in range(1, 4):
params = {"page": page, "limit": 50}
response = requests.get(url, params=params)
print(response.json())
Example 3: Filtering Data Using Parameters
Fetch filtered product listings:
params = {"category": "electronics", "brand": "XYZ"}
response = requests.get(url, params=params)
print(response.json())
Conclusion
Passing parameters into a URL with Python’s requests
library is a powerful yet straightforward process. From understanding query strings to encoding special characters, mastering this skill enhances your ability to interact with APIs effectively. Experiment with the examples provided and share your thoughts or additional use cases in the comments below!